POOPOO: 배변 일기 앱
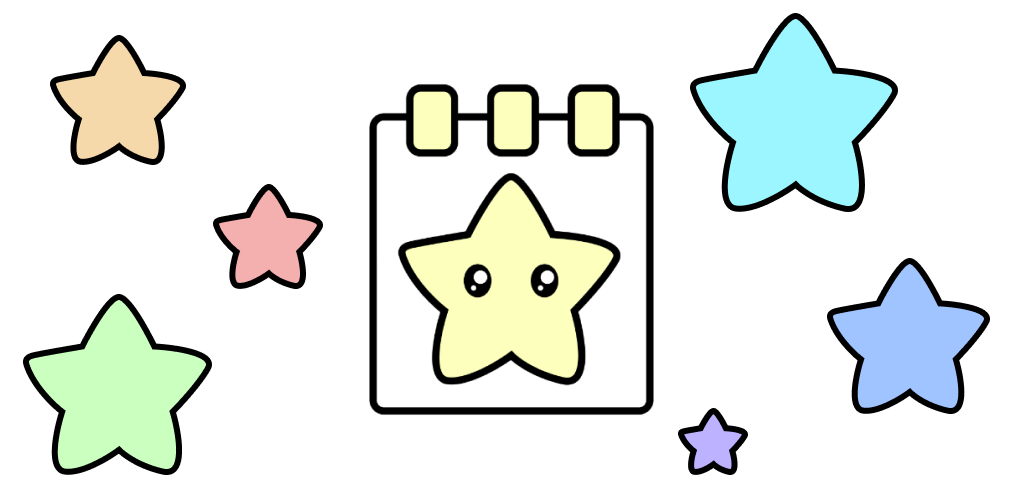
SMALL
행동패턴 목록
- 책임연쇄 패턴 (Chan of Responsibility Pattern)
- 커맨드 패턴 (Command Pattern)
- 해석자 패턴 (Interpreter Pattern)
- 반복자 패턴 (Iterator Pattern)
- 중재자 패턴 (Mediator Pattern)
- 메멘토 패턴 (Memento Pattern)
- 관찰자 패턴 (Observer Pattern)
- 상태 패턴 (State Pattern)
- 전략 패턴 (Strategy Pattern)
- 템플릿 패턴 (Template Pattern)
객체의 동작이 상태에 따라 변하는 경우에 사용한다. 옵저버패턴과의 차이를 생각해보고 적재적소에 사용해야 할 것 같다. 관찰자 패턴은 상태가 변경시에 그때그때 알려주는 패턴이고 상태패턴은 상태에 따라 처리를 다르게 적용하는 패턴이기 때문에 차이가 있다.
장점
- 상태별 동작을 유지합니다.
- 상태 전이를 명시적으로 만듭니다.
사용시기
- 객체의 동작이 상태에 의존하고 새로운 상태에 따라 런타임에 동작을 변경할 수 있어야 하는 경우.
- 조작에 오브젝트의 상태에 따라 큰 여러 부분으로 된 조건문이 있는 경우에 사용됩니다.
UML
State Interface Class : State
public interface State {
public void open();
public void close();
public void log();
public void update();
}
State Class : Accounting, Sales, Management
public class Accounting implements State {
@Override
public void open() {
System.out.println("open database for accounting");
}
@Override
public void close() {
System.out.println("close the database");
}
@Override
public void log() {
System.out.println("log activities");
}
@Override
public void update() {
System.out.println("Accounting has been updated");
}
}
public class Sales implements State {
@Override
public void open() {
System.out.println("open database for sales");
}
@Override
public void close() {
System.out.println("close the database");
}
@Override
public void log() {
System.out.println("log activities");
}
@Override
public void update() {
System.out.println("Sales has been updated");
}
}
public class Management implements State {
@Override
public void open() {
System.out.println("open database for Management");
}
@Override
public void close() {
System.out.println("close the database");
}
@Override
public void log() {
System.out.println("log activities");
}
@Override
public void update() {
System.out.println("Management has been updated");
}
}
Operation Class : Controller, StatePatternDemo
public class Controller {
public static Accounting accounting;
public static Sales sales;
public static Management management;
private static State state;
Controller() {
accounting = new Accounting();
sales = new Sales();
management = new Management();
}
public void setAccountingState() {
state = accounting;
}
public void setSalesState() {
state = sales;
}
public void setManagementState() {
state = management;
}
public void open() {
state.open();
}
public void close() {
state.close();
}
public void log() {
state.log();
}
public void update() {
state.update();
}
}
public class StatePatternDemo {
Controller controller;
StatePatternDemo(String state) {
controller = new Controller();
if(state.equalsIgnoreCase("management"))
controller.setManagementState();
if(state.equalsIgnoreCase("sales"))
controller.setSalesState();
if(state.equalsIgnoreCase("accounting"))
controller.setAccountingState();
controller.open();
controller.log();
controller.close();
controller.update();
}
public static void main(String args[]) {
new StatePatternDemo("management");
}
}
출처 : https://www.javatpoint.com/
Tutorials - Javatpoint
Tutorials, Free Online Tutorials, Javatpoint provides tutorials and interview questions of all technology like java tutorial, android, java frameworks, javascript, ajax, core java, sql, python, php, c language etc. for beginners and professionals.
www.javatpoint.com
LIST