POOPOO: 배변 일기 앱
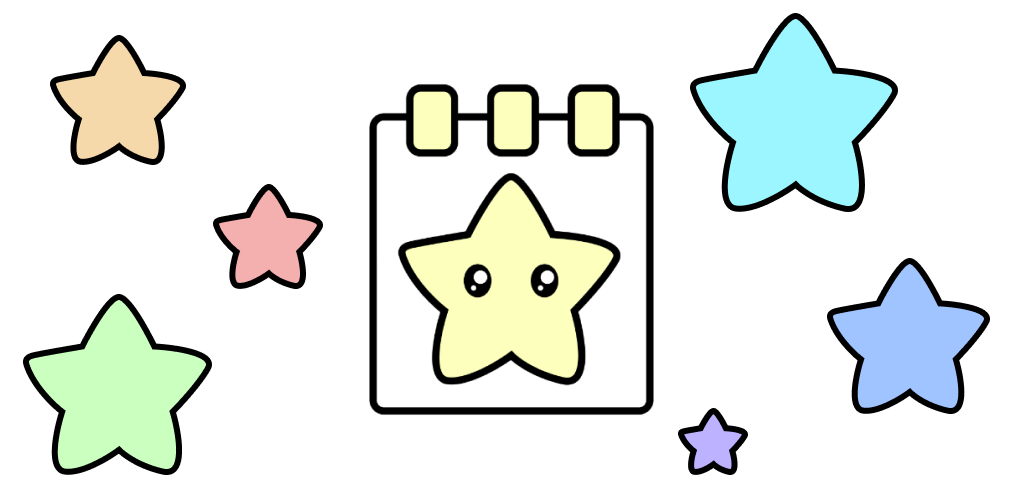
행동패턴 목록
- 책임연쇄 패턴 (Chan of Responsibility Pattern)
- 커맨드 패턴 (Command Pattern)
- 해석자 패턴 (Interpreter Pattern)
- 반복자 패턴 (Iterator Pattern)
- 중재자 패턴 (Mediator Pattern)
- 메멘토 패턴 (Memento Pattern)
- 관찰자 패턴 (Observer Pattern)
- 상태 패턴 (State Pattern)
- 전략 패턴 (Strategy Pattern)
- 템플릿 패턴 (Template Pattern)
커맨드를 추가하고 난 다음 Receiver, Invoker 클래스만 수정하기만 하면 바로 추가된 커맨드를 사용할 수 있다. 지금은 이 커맨드라는 부분이 어떤 장치에 들어가는 프로그램에 사용하는 경우에 좀 더 적합한 것 같다.
장점
- 조작을 호출하는 객체를 실제로 조작을 수행하는 객체와 분리합니다.
- 기존 클래스는 그대로 유지되므로 새 명령을 쉽게 추가할 수 있습니다.
사용시기
- 작업 수행에 따라 객체를 매개변수화 해야하는 경우
- 다른 시간에 요청을 작성하고 실행해야하는 경우
- 롤백, 로깅 또는 트랜잭션 기능을 지원해야 할 때
UML
Receiver Class : Document
- 수행할 작업들을 알고 있는 클래스입니다.
public class Document {
public void open(){
System.out.println("Document Opened");
}
public void save(){
System.out.println("Document Saved");
}
}
Command Class : ActionListenerCommand
- 작업을 실행하기 위한 인터페이스 입니다.
public interface ActionListenerCommand {
public void execute();
}
ConcreteCommand Class : ActionSave, ActionOpen
- 커맨드 클래스를 확장하고 execute 메소드를 구현합니다.
- 이 클래스는 action과 receiver 사이에 바인딩을 작성합니다.
public class ActionSave implements ActionListenerCommand{
private Document doc;
public ActionSave(Document doc) {
this.doc = doc;
}
@Override
public void execute() {
doc.save();
}
}
public class ActionOpen implements ActionListenerCommand{
private Document doc;
public ActionOpen(Document doc) {
this.doc = doc;
}
@Override
public void execute() {
doc.open();
}
}
Invoker Class : MenuOptions
- 명령을 받아서 수행합니다.
public class MenuOptions {
private ActionListenerCommand openCommand;
private ActionListenerCommand saveCommand;
public MenuOptions(ActionListenerCommand open, ActionListenerCommand save) {
this.openCommand = open;
this.saveCommand = save;
}
public void clickOpen(){
openCommand.execute();
}
public void clickSave(){
saveCommand.execute();
}
}
Operation Class : CommandPatternClient
- ConcreteCommand 클래스를 생성하고 Receiver를 연결합니다.
public class CommandPatternClient {
public static void main(String[] args) {
Document doc = new Document();
ActionListenerCommand clickOpen = new ActionOpen(doc);
ActionListenerCommand clickSave = new ActionSave(doc);
MenuOptions menu = new MenuOptions(clickOpen, clickSave);
menu.clickOpen();
menu.clickSave();
}
}
출처 : https://www.javatpoint.com/
Tutorials - Javatpoint
Tutorials, Free Online Tutorials, Javatpoint provides tutorials and interview questions of all technology like java tutorial, android, java frameworks, javascript, ajax, core java, sql, python, php, c language etc. for beginners and professionals.
www.javatpoint.com