POOPOO: 배변 일기 앱
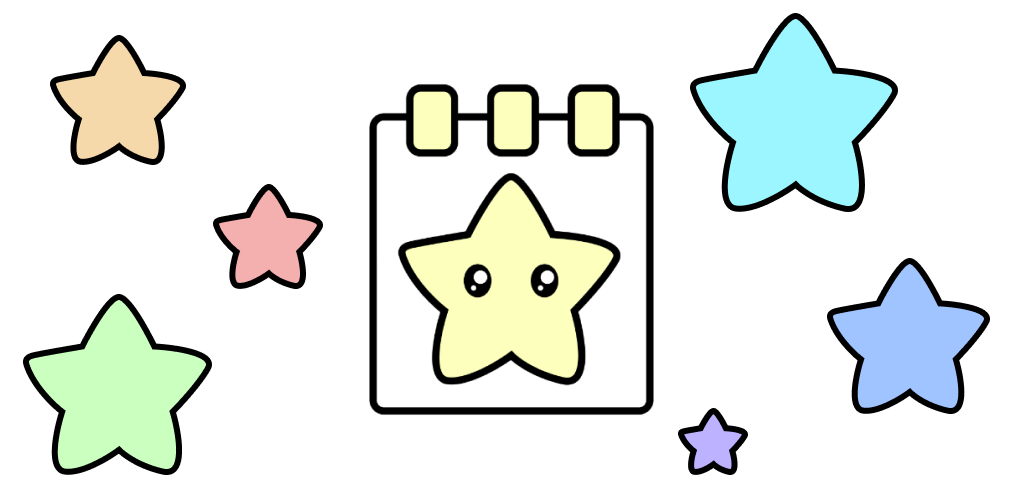
SMALL
구조패턴 목록
- 어댑터 패턴 (Adapter Pattern)
- 브릿지 패턴 (Bridge Pattern)
- 컴포짓 패턴 (Composite Pattern)
- 데코레이터 패턴 (Decorator Pattern)
- 퍼사드 패턴 (Facade Pattern)
- 플라이웨이트 패턴 (Flyweight Pattern)
- 프록시 패턴 (Proxy Pattern)
게임이나 지도처럼 동일한 객체를 특정 속성만 바꿔서 재사용이 가능한 경우에 적용하면 큰 효과를 기대할 수 있는 패턴인 것 같다.
장점
- 객체의 수를 줄입니다.
- 객체가 지속되는 경우에 필요한 메모리 및 저장장치의 양을 줄입니다.
사용시기
- 응용프로그램이 많은 수의 객체를 사용하는 경우
- 물체의 수량으로 인해 저장비용이 높은 경우
- 응용프로그램이 객체 ID에 의존하지 않는 경우
UML
Model Class : Node, CircleNode
public interface Node {
public void draw();
}
public class CircleNode implements Node {
private String color;
private int x;
private int y;
private int radius;
public Circle(String color){
this.color = color;
}
public void setX(int x) {
this.x = x;
}
public void setY(int y) {
this.y = y;
}
public void setRadius(int radius) {
this.radius = radius;
}
@Override
public void draw() {
System.out.println("CircleNode - Color : " + color);
System.out.println("CircleNode - X : " + x);
System.out.println("CircleNode - Y : " + y);
System.out.println("CircleNode - Radius : " + radius);
}
}
Factory Class : NodeFactory
public class NodeFactory {
private static final HashMap<String, Node> circleMap = new HashMap();
public static Node getCircleNode(String color) {
CircleNode circleNode = (CircleNode)circleNodeMap.get(color);
if(circleNode == null) {
circleNode = new CircleNode(color);
circleNodeMap.put(color, circle);
System.out.println("CircleNode 생성 : " + color);
}
return circle;
}
}
Operation Class : FlyWeightPatternDemo
public class FlyweightPatternDemo {
private static final String colors[] = { "Red", "Green", "Blue", "White", "Black" };
public static void main(String[] args) {
for(int i=0; i < 20; ++i) {
String randomColor = colors[(int)(Math.random()*colors.length)];
int randomX = (int)(Math.random()*100);
int randomY = (int)(Math.random()*100);
int radius = 100;
CircleNode circleNode = (CircleNode)NodeFactory.getCircleNode(randomColor);
circleNode.setX(randomX);
circleNode.setY(randomY);
circleNode.setRadius(radius);
circleNode.draw();
}
}
}
출처 : https://www.javatpoint.com/
Tutorials - Javatpoint
Tutorials, Free Online Tutorials, Javatpoint provides tutorials and interview questions of all technology like java tutorial, android, java frameworks, javascript, ajax, core java, sql, python, php, c language etc. for beginners and professionals.
www.javatpoint.com
LIST