POOPOO: 배변 일기 앱
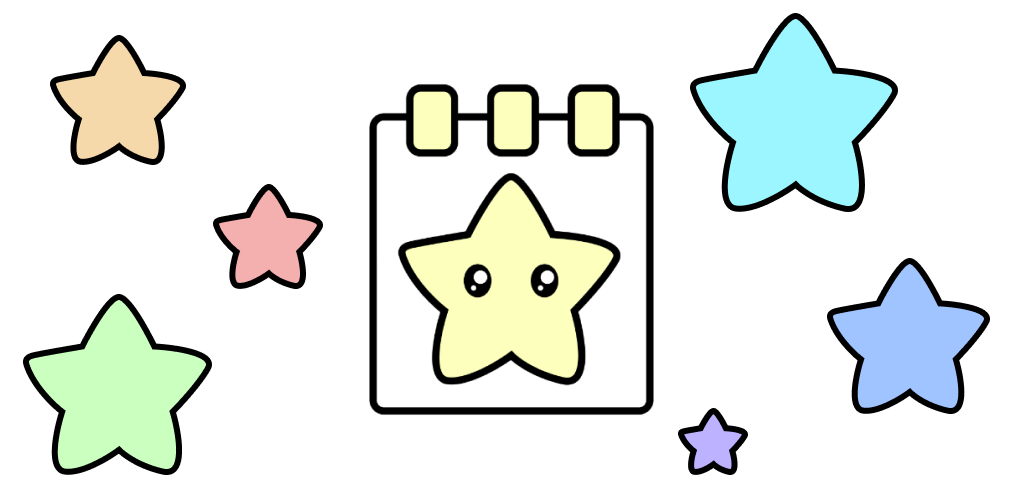
구조패턴 목록
- 어댑터 패턴 (Adapter Pattern)
- 브릿지 패턴 (Bridge Pattern)
- 컴포짓 패턴 (Composite Pattern)
- 데코레이터 패턴 (Decorator Pattern)
- 퍼사드 패턴 (Facade Pattern)
- 플라이웨이트 패턴 (Flyweight Pattern)
- 프록시 패턴 (Proxy Pattern)
객체와 객체 사이의 연관관계가 계층으로 표현해야 하고, 같은 계층에서 다른 역할을 구현할 필요가 있다면 이 패턴이 필요할 것 같다.
장점
- 기본적이고 복잡한 객체를 포함하는 클래스 계층을 정의합니다.
- 새로운 종류의 구성 요소를 쉽게 추가할 수 있습니다.
- 관리 가능한 클래스 또는 인터페이스로 구조의 유연성을 제공합니다.
사용법
- 객체의 전체 또는 부분 계층을 나타내려는 경우
- 다른 개체에 영향을 주지 않고 개별 개체에 책임을 동적으로 추가해야하는 경우
UML
Component Class : Employee
- 컴포지션의 객체에 대한 인터페이스를 선언합니다
- 모든 클래스에 공통인 인터페이스의 기본동작을 적절하게 구현합니다
- 자식 구성요소에 엑세스하고 관리하기위한 인터페이스를 선언합니다
public interface Employee {
public int getId();
public String getName();
public double getSalary();
public void print();
public void add(Employee employee);
public void remove(Employee employee);
public Employee getChild(int i);
}
Leaf Class : Accountant, Cashier
- 자식 구성요소가 없습니다
- 컴포지션에서 리프객체를 나타냅니다
- 컴포지션에서 기본 객체의 동작을 정의합니다
public class Accountant implements Employee{
private int id;
private String name;
private double salary;
public Accountant(int id,String name,double salary) {
this.id=id;
this.name = name;
this.salary = salary;
}
@Override
public void add(Employee employee) {}
@Override
public Employee getChild(int i) {return null;}
@Override
public int getId() {
return id;
}
@Override
public String getName() {
return name;
}
@Override
public double getSalary() {
return salary;
}
@Override
public void print() {
System.out.println("=========================");
System.out.println("Id ="+getId());
System.out.println("Name ="+getName());
System.out.println("Salary ="+getSalary());
System.out.println("=========================");
}
@Override
public void remove(Employee employee) {}
}
public class Cashier implements Employee{
private int id;
private String name;
private double salary;
public Cashier(int id,String name,double salary) {
this.id=id;
this.name = name;
this.salary = salary;
}
@Override
public void add(Employee employee) {}
@Override
public Employee getChild(int i) {return null;}
@Override
public int getId() {
return id;
}
@Override
public String getName() {
return name;
}
@Override
public double getSalary() {
return salary;
}
@Override
public void print() {
System.out.println("=========================");
System.out.println("Id ="+getId());
System.out.println("Name ="+getName());
System.out.println("Salary ="+getSalary());
System.out.println("=========================");
}
@Override
public void remove(Employee employee) {}
}
Composite Class : BankManager
- 자식 구성요소가 존재합니다
- 컴포넌트 인터페이스에서 하위 관련 조작을 구현합니다
public class BankManager implements Employee {
private int id;
private String name;
private double salary;
List<Employee> employees = new ArrayList<Employee>();
public BankManager(int id,String name,double salary) {
this.id=id;
this.name = name;
this.salary = salary;
}
@Override
public void add(Employee employee) {
employees.add(employee);
}
@Override
public Employee getChild(int i) {
return employees.get(i);
}
@Override
public void remove(Employee employee) {
employees.remove(employee);
}
@Override
public int getId() {
return id;
}
@Override
public String getName() {
return name;
}
@Override
public double getSalary() {
return salary;
}
@Override
public void print() {
System.out.println("==========================");
System.out.println("Id ="+getId());
System.out.println("Name ="+getName());
System.out.println("Salary ="+getSalary());
System.out.println("==========================");
Iterator<Employee> it = employees.iterator();
while(it.hasNext()) {
Employee employee = it.next();
employee.print();
}
}
}
Operation Class : CompositePatternDemo
- 컴포넌트 인터페이스를 통해 컴포지션의 객체를 조작합니다
public class CompositePatternDemo {
public static void main(String args[]){
Employee emp1=new Cashier(101,"Sohan Kumar", 20000.0);
Employee emp2=new Cashier(102,"Mohan Kumar", 25000.0);
Employee emp3=new Accountant(103,"Seema Mahiwal", 30000.0);
Employee manager1=new BankManager(100,"Ashwani Rajput",100000.0);
manager1.add(emp1);
manager1.add(emp2);
manager1.add(emp3);
manager1.print();
}
}
출처 : https://www.javatpoint.com/
Tutorials - Javatpoint
Tutorials, Free Online Tutorials, Javatpoint provides tutorials and interview questions of all technology like java tutorial, android, java frameworks, javascript, ajax, core java, sql, python, php, c language etc. for beginners and professionals.
www.javatpoint.com